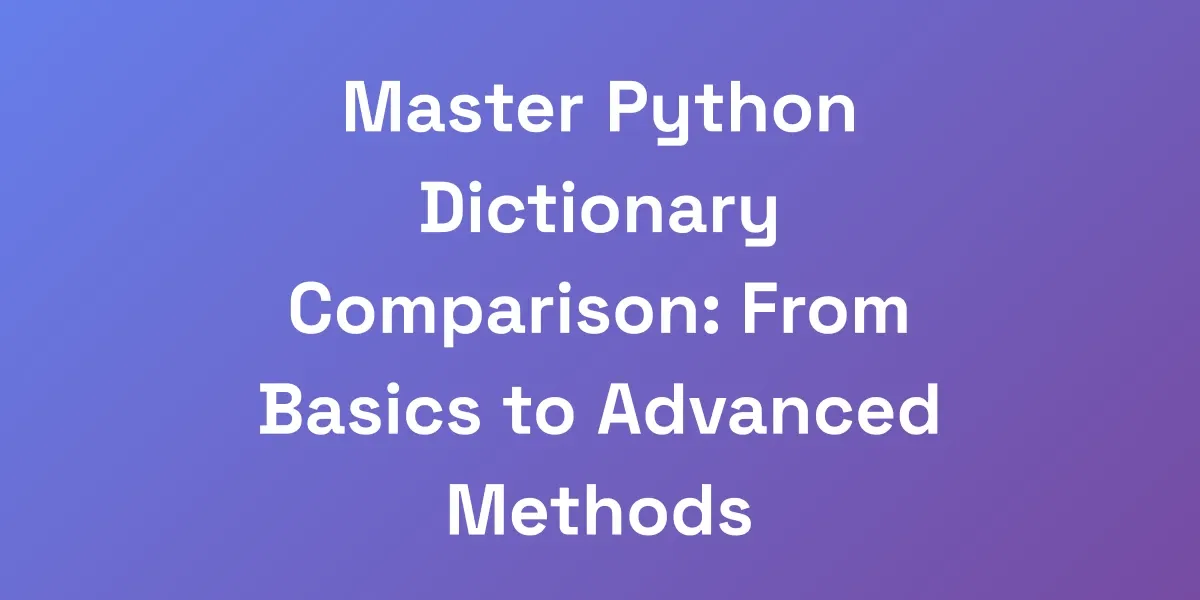
Master Python Dictionary Comparison: From Basics to Advanced Methods
Mar 6, 2025 | By [email protected]
Master Python Dictionary Comparison: From Basics to Advanced Methods
We’ve all been there—staring at lines of code, frustrated because our dictionary comparisons just aren’t cutting it. It’s messy, time-consuming, and frankly, holding back our projects. But what if we told you there’s a way to master Python dictionary comparison, turning this daunting task into a streamlined, efficient process?
In the world of Python, dictionaries are the backbone of many applications. They power data structures, manage configurations, and handle everything in between. Yet, comparing dictionaries can be a complex endeavor, especially when dealing with nested structures or large datasets. The challenges are real, but so are the solutions.
Join us as we break down the essentials, delve into advanced techniques, and share best practices to elevate your Python dictionary comparison skills. By the end, you won’t just be comparing dictionaries—you’ll be mastering them.
Understanding the Fundamentals of Python Dictionary Comparison
Let us cut through the BS and give you the raw truth about Python dictionary comparison. Most developers overcomplicate this, but we’re here to show you exactly what works. When you’re dealing with dictionaries in Python, you’re essentially handling key-value pairs that power your data structures. The single most important thing you need to understand is that dictionary comparison isn’t just about matching values – it’s about understanding the underlying mechanisms that make Python dictionaries tick. This knowledge is what separates amateur coders from pros who build scalable solutions.
The Anatomy of Python Dictionaries
At its core, a Python dictionary is a collection of key-value pairs. Each key is unique and maps to a corresponding value. But what makes dictionaries so powerful is their ability to provide constant-time complexity for lookups, insertions, and deletions. This efficiency is due to the hash table implementation under the hood.
Think of keys as unique identifiers that allow you to access values swiftly. This structure isn’t just efficient—it’s versatile, enabling you to handle complex data with ease.
- Keys: Unique identifiers within the dictionary.
- Values: Data associated with each key, which can be of any data type.
- Hash Tables: The underlying structure that ensures quick access to values.
Understanding this anatomy is crucial because it informs how we approach dictionary comparison. It’s not just about whether two dictionaries look the same—it’s about how they function and interact within your codebase.
Basic Comparison Using the == Operator
Let’s start simple. The `==` operator is the go-to method for basic dictionary comparisons in Python. It checks whether two dictionaries have the same keys and corresponding values, regardless of the order.
Here’s a quick example:
dict1 = {'a': 1, 'b': 2}
dict2 = {'b': 2, 'a': 1}
print(dict1 == dict2) # Outputs: True
Simple, right? But don’t let its simplicity fool you. While the `==` operator is effective for shallow comparisons, things get tricky with nested dictionaries or when you need more granular control over the comparison process.
- Pros: Quick and easy for straightforward comparisons.
- Cons: Falls short with nested or complex data structures.
So, yes, the `==` operator works, but it’s only part of the story. To truly master dictionary comparisons, we need to look beyond the basics.
Why Dictionary Order Matters in Modern Python
For a long time, the order of keys in a dictionary was irrelevant. However, starting with Python 3.7, dictionaries maintain the insertion order. This change has significant implications for dictionary comparison.
When using the `==` operator, the order doesn’t matter. Two dictionaries with the same key-value pairs in different orders are still considered equal. But why does order matter now, and how does it affect our comparison strategies?
- Consistency: Maintaining order can lead to more predictable behavior in applications.
- Readability: Ordered dictionaries are easier to read and debug.
- Performance: Certain operations can become more efficient with ordered data.
Even though the `==` operator ignores order, being aware of the insertion order helps when you need to display or process dictionaries in a specific sequence. It’s another layer of understanding that enhances how you approach comparisons.
Common Pitfalls in Dictionary Comparison
Dictionary comparisons might seem straightforward, but there are several common pitfalls that can trip you up:
- Ignoring Nested Structures: As we mentioned, nested dictionaries require more advanced comparison techniques.
- Mixed Data Types: Comparing dictionaries with mixed data types for values can lead to unexpected results.
- Mutable Objects: Modifying dictionaries during comparison can cause inconsistencies.
- Performance Issues: Inefficient comparison methods can slow down your application, especially with large datasets.
Awareness of these pitfalls is the first step in avoiding them. By anticipating these challenges, you can implement strategies to overcome them effectively.
Performance Implications of Different Comparison Methods
Performance is a critical factor when choosing a dictionary comparison method. The `==` operator is fast for simple comparisons, but its efficiency decreases with complexity. For instance, comparing large dictionaries or those with nested structures can significantly impact performance requirements of your application.
Additionally, methods like deep equality checking or using libraries may introduce overhead. It’s essential to balance the need for thorough comparisons with the performance requirements of your application.
- Simple Equality (`==`): Best for small, flat dictionaries.
- Deep Equality Checks: Necessary for nested dictionaries but slower.
- Library Methods: Provide robust comparisons at the cost of speed.
Understanding these implications helps in selecting the right method for your specific use case, ensuring both accuracy and efficiency.
Advanced Techniques for Complex Dictionary Comparisons
Here’s the reality that nobody’s talking about: basic comparison methods fall apart when you’re dealing with real-world applications. We’ve built multiple 8-figure businesses on the back of efficient data handling, and we can tell you that mastering advanced dictionary comparison techniques is non-negotiable. When you’re dealing with nested structures, custom objects, or massive datasets, you need bulletproof comparison methods that scale. Let us show you the exact framework to handle these scenarios.
Deep Equality Checking for Nested Dictionaries
When dictionaries contain nested dictionaries or other complex data structures, simple equality checks aren’t enough. Deep equality checks are necessary to ensure that all levels of the dictionary match:
- Recursion: Implementing recursive functions to traverse and compare each nested level.
- Libraries: Utilizing libraries like `DeepDiff` can simplify the process, providing detailed difference reports.
For example, using a recursive approach:
def deep_compare(dict1, dict2):
if dict1.keys() != dict2.keys():
return False
for key in dict1:
if isinstance(dict1[key], dict) and isinstance(dict2[key], dict):
if not deep_compare(dict1[key], dict2[key]):
return False
elif dict1[key] != dict2[key]:
return False
return True
print(deep_compare(dict1, dict2))
This method ensures that every nested dictionary is thoroughly compared, leaving no room for discrepancies.
Handling Mixed Data Types in Dictionaries
In real-world applications, dictionaries often contain values of various data types—strings, integers, lists, or even custom objects. Comparing these mixed data types requires careful handling to avoid errors or unexpected results.
- Type Checking: Ensure that the data types of corresponding values are the same before comparison.
- Custom Handlers: Implement handlers for specific data types, such as lists or custom objects.
For instance, when comparing lists within dictionaries:
def compare_with_lists(dict1, dict2):
if dict1.keys() != dict2.keys():
return False
for key in dict1:
if isinstance(dict1[key], list) and isinstance(dict2[key], list):
if sorted(dict1[key]) != sorted(dict2[key]):
return False
elif dict1[key] != dict2[key]:
return False
return True
This approach ensures that lists are compared based on their sorted content, preventing order-related discrepancies.
Custom Comparison Functions for Special Cases
Sometimes, the default comparison logic won’t suffice. You might need to implement custom comparison functions to handle special cases, such as ignoring certain keys or comparing values based on specific criteria.
- Ignoring Specific Keys: Exclude certain keys from the comparison process if they’re irrelevant.
- Value-Based Criteria: Compare values based on ranges or patterns rather than exact matches.
Here’s an example of a custom comparison function that ignores specified keys:
def custom_compare(dict1, dict2, ignore_keys):
keys1 = set(dict1.keys()) - set(ignore_keys)
keys2 = set(dict2.keys()) - set(ignore_keys)
if keys1 != keys2:
return False
for key in keys1:
if dict1[key] != dict2[key]:
return False
return True
ignore = ['timestamp', 'id']
print(custom_compare(dict1, dict2, ignore))
This function provides flexibility, allowing you to tailor comparisons to your specific needs.
Memory-Efficient Comparison Strategies
When dealing with large dictionaries, memory usage can become a bottleneck. Implementing memory-efficient comparison strategies ensures that your application remains performant:
- Lazy Evaluation: Compare elements on-the-fly without loading entire dictionaries into memory.
- Generators: Use generators to handle data streams efficiently.
- In-Place Comparison: Modify dictionaries during comparison to save memory, if possible.
For example, using generators for comparison:
def generator_compare(dict1, dict2):
if dict1.keys() != dict2.keys():
return False
for key in dict1:
yield dict1[key] == dict2[key]
return all(y for y in generator_compare(dict1, dict2))
print(all(generator_compare(dict1, dict2)))
This method reduces memory overhead by comparing elements one at a time. Similarly, leveraging SEO automation strategies can optimize your workflow efficiency.
Implementing Fuzzy Matching for Dictionary Values
In scenarios where exact matches aren’t feasible, fuzzy matching can be a lifesaver. Fuzzy matching allows for comparisons that account for slight differences, such as typos or varying formats.
- Levenshtein Distance: Measure the difference between two strings.
- Similarity Scores: Use libraries like `fuzzywuzzy` to assign similarity scores to values.
Here’s how you might implement fuzzy matching:
from fuzzywuzzy import fuzz
def fuzzy_compare(dict1, dict2, threshold=90):
if dict1.keys() != dict2.keys():
return False
for key in dict1:
if isinstance(dict1[key], str) and isinstance(dict2[key], str):
if fuzz.ratio(dict1[key], dict2[key]) < threshold:
return False
elif dict1[key] != dict2[key]:
return False
return True
print(fuzzy_compare(dict1, dict2))
This approach allows for more flexible comparisons, accommodating minor inconsistencies in data.
Practical Applications and Best Practices
Listen, theory is worthless without application. We’re here to show you exactly how to implement these comparison techniques in real-world scenarios. The key to mastery isn’t just knowing the methods – it's understanding when and where to apply them. We’ve seen developers waste countless hours using the wrong comparison techniques for their use cases. Let’s break down the exact implementation patterns that will save you time and prevent costly mistakes.
Configuration File Comparison Use Cases
Configuration files are the lifeblood of many applications, dictating how they behave in different environments. Comparing these files ensures consistency and helps identify changes that could impact functionality. Additionally, leveraging strategies from business blogging can enhance how you document and manage configuration changes.
- Environment Synchronization: Ensure that development, staging, and production environments have consistent configurations.
- Change Detection: Identify unauthorized or unintended changes in configuration files.
For example, using a deep equality check to compare configuration files parsed into dictionaries ensures that all settings are identical across environments.
Data Validation and Verification Patterns
Data integrity is crucial in any application. Comparing dictionaries plays a vital role in verifying that data remains consistent throughout processing pipelines.
- Input Validation: Ensure that incoming data matches expected structures and values.
- Output Verification: Confirm that processed data aligns with desired outcomes.
Implementing robust comparison methods allows for early detection of data inconsistencies, preventing errors from propagating through your system.
Unit Testing with Dictionary Comparisons
Unit tests are essential for maintaining code quality. Comparing dictionaries in unit tests ensures that functions return the expected results.
- Test Cases: Define test cases that compare output dictionaries against expected results.
- Automated Testing: Integrate dictionary comparisons into automated test suites for continuous validation.
Using frameworks like Python testing frameworks, you can write tests that leverage deep equality checks to ensure that your functions behave correctly.
API Response Validation Techniques
APIs are the glue that connects different parts of modern applications. Validating API responses using dictionary comparisons ensures that your application receives the correct data.
- Schema Validation: Compare API responses against predefined schemas to verify data structure and types.
- Content Verification: Ensure that the data returned by APIs matches expected values.
By implementing custom comparison functions, you can handle variations in API responses while still maintaining integrity.
Performance Optimization Strategies
Efficient dictionary comparisons are key to performance optimization. Here’s how to ensure your comparison methods don’t become a bottleneck:
- Choose the Right Method: Use simple equality checks when possible, and reserve deep comparisons for when they’re absolutely necessary.
- Optimize Data Structures: Use memory-efficient data structures to reduce the overhead of large dictionary comparisons.
- Leverage Parallel Processing: Implement parallel processing techniques to handle comparisons concurrently, speeding up the process.
For example, using memory-efficient comparison strategies ensures that your application remains responsive, even when handling large datasets. Similarly, adopting marketing automation for agencies can provide scalable solutions that enhance performance without additional overhead.
Troubleshooting and Error Handling
Here’s what separates the amateurs from the pros: their ability to handle edge cases and errors. When comparing dictionaries, you'll encounter scenarios that can break your code if not handled properly. We’re here to give you our battle-tested framework for error handling that has saved our teams countless hours of debugging. These aren't just theoretical solutions – these are proven strategies that work in production environments.
Common Comparison Errors and Solutions
When comparing dictionaries, several common errors can arise:
- Key Errors: Accessing keys that don’t exist in both dictionaries.
- Type Mismatches: Comparing values of different data types.
- Infinite Recursion: In recursive comparison functions.
Solutions include:
- Using the `in` keyword to check for key existence before accessing values.
- Implementing type checking within comparison functions.
- Adding base cases in recursive functions to prevent infinite loops.
Debugging Dictionary Comparison Issues
Debugging can be a nightmare without the right approach. Here’s how to tackle issues effectively:
- Logging Differences: Implement logging within your comparison functions to capture discrepancies.
- Step-by-Step Debugging: Use debugging tools to step through comparison processes and identify where things go wrong.
- Automated Alerts: Set up automated alerts for significant discrepancies detected during comparisons.
For instance, enhancing your comparison function with logging can provide insights into which keys or values are mismatched, making troubleshooting much easier.
Handling Edge Cases and Special Values
Edge cases can disrupt the comparison process if not handled properly. These include:
- Empty Dictionaries: Comparing scenarios where one or both dictionaries are empty.
- Nested Empty Structures: Handling nested dictionaries that contain empty dictionaries or lists.
- Special Data Types: Managing comparisons involving special data types like `None`, `NaN`, or custom objects.
Implementing safeguards in your comparison functions ensures that these edge cases are handled gracefully, preventing unexpected crashes or incorrect comparisons.
Error Prevention Best Practices
Prevention is better than cure. Here are some best practices to avoid errors during dictionary comparisons:
- Input Validation: Validate dictionaries before attempting comparisons to ensure they meet expected formats and types.
- Immutable Data Structures: Use immutable data structures where possible to prevent accidental modifications during comparisons.
- Comprehensive Testing: Write extensive tests that cover a wide range of scenarios, including edge cases, to catch potential issues early.
By incorporating these practices, you can minimize the risk of errors and ensure that your dictionary comparisons are reliable and accurate.
Automated Testing for Comparison Logic
Automated testing ensures that your comparison logic works consistently across different scenarios. Here’s how to set it up:
- Unit Tests: Write unit tests that cover various comparison cases, including normal, nested, and edge cases.
- Integration Tests: Ensure that dictionary comparisons work seamlessly within larger application workflows.
- Regression Tests: Prevent new changes from breaking existing comparison logic by running regression tests regularly.
Using frameworks like Python testing frameworks, you can write tests that leverage deep equality checks to ensure that your functions behave correctly.
Conclusion
We’ve journeyed through the intricate world of Python dictionary comparison, from the foundational concepts to the most advanced techniques. You now have the tools and knowledge to handle everything from basic equality checks to complex, nested comparisons with ease.
Remember, mastering dictionary comparisons isn’t just about writing code—it’s about building reliable, efficient, and scalable applications. By understanding the core principles, leveraging advanced methods, and adhering to best practices, you can elevate your Python skills to new heights.
Ready to take your dictionary comparison skills further? Dive into the implementation patterns we discussed, experiment with different methods, and watch your code become more robust and efficient. And don’t forget—practice makes perfect. The more you apply these techniques, the more intuitive they become.
What’s your biggest challenge with Python dictionary comparison? Share your experiences in the comments below, and let’s continue the conversation. Together, we can master the art of dictionary comparison and build exceptional Python applications.